Portraits
I’m starting to test portrait integration into NPC talkers. Here’s an in-game mockup.
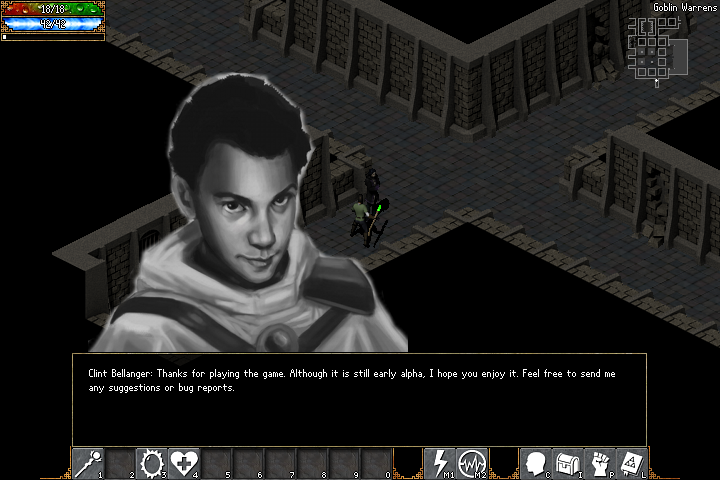
Campaign Dialog
I’ve been pondering how to handle dialog that depends on the current state of the story. The NPC files will have [dialog] blocks that contain story-dependent conversations.
Here’s an example of plain back and forth dialog that only occurs once (sets a new campaign status upon completion).
[dialog]
requires_status=1
him=Welcome to … say, you wouldn’t happen to be Matthias’s kid?
you=I didn’t know my father was known in this part of the Kingdom. My name’s %n.
him=The kingdom’s smaller than you think. I’m Reginald. I worked with your old man on the Skybridge project, back before your time. How is he these days?
you=Surviving, like the rest of us.
him=Glad to hear it. Say, unless you’re just passing through, pay a visit to Jameson over at the Frothy Tankard Inn. He served at Skybridge with us too. The two of them were best of friends. Hells, Jameson might even put you up for the night free of charge.
set_status=2
If you talk to the guy again, he’ll say something different. The engine will display the lattermost dialog option where the requirements are all met.
[dialog]
requires_status=2
him=Frothy Tankard Inn is right on town square. This road will take you straight to it. I’m sure Jameson will have plenty of stories to share about your father.
Now here’s an example of how quests might work with dialogs.
[dialog]
requires_status=10
her=There is a storage room under the cathedral ruins. There should at least be a few sacks of grains, if the plague rats haven’t gotten to them first. It would buy us a couple more weeks here… but don’t go risking your neck on our account.
set_status=11
[dialog]
requires_status=11
her=There must be a safe path to the cathedral storage rooms…
Campaign status checks could also be added to map events.
[event]
# if on the “sack of grain” quest, drop it here
requires_status=11
requires_not=12
msg=You find a sack of wheat grain. Maybe luck has turned around for those refugees.
loot=item,2000
set_status=12
And quest turn-in:
[dialog]
requires_status=12
requires_item=2000
her=You’ve found food! I don’t know what to say, or how we could possibly repay you.
remove_item=2000
reward_xp=100
set_status=13
So, the possible fields in a dialog node:
- requires_status
- requires_item
- requires_not
- him
- her
- you
- remove_item
- reward_item
- reward_xp
- reward_gold
- set_status
Campaign statuses will be checked or set in various ways to create stories and quests.
- Map Events might only occur on a specific status, or set a status
- Enemies might only spawn on a specific status, or set a status when defeated
- The Log menu will display reminder text based on current statuses
Examples of the kind of simple connections possible with this system:
- Boss treasure chest only openable if the boss is dead
- Story bosses that stay dead
- Conditional map teleports to make map phasing (e.g. village burns down, next time you enter that area it actually loads a different map)
- Doors that require specific keys to open
- Chest drops a quest item if you’re on the quest, otherwise it drops random loot
The status booleans will be written to the save file. The first implementation will have 1024 status fields. I’ll store them as plain text hexadecimal like this:
campaign=f1fea3b000000000000000000000000000000000000000000000000000000000